Welcome back to our series on LINQ in .NET! In the first part, we introduced the basics of LINQ, including its syntax and structure. We saw a couple of examples — selecting all even numbers out of a list of numbers; selecting all the students over the age of 18 from a list of Student objects. We learned that by using LINQ, we get a consistent paradigm for interacting with datasets in .NET across a wide range of data types, whether that’s a database query or an XML document or an assortment of in-memory collections. it helps us elminate the noise associated with traditional looping constructs such as the for and foreach loops.
What Can I Use LINQ For?
There is a rich library of LINQ operators (a lot of which we’ll look at it in this series) that allows you to deal with data in elegant ways.
- Selecting data. We’ll look at some exampels of this later in this post.
- Change the shape of your data from one form to another.
- Find data using a
Where
clause, much like you would do with SQL. - Target elements easily – first item in a collection, last item in a collection, single item in a collection etc.
- Organize data by sorting them in ascending or descending order.
- We can segment data by skipping records and taking records.
- You can iterate over data but in a more succint way than traditional looping structures.
- You can run various checks against your datasets to see if certain criteria is met or not met.
- You can compare two sets to see what’s the same or what’s different between them.
- We can join two data sets into one.
- We can extract distinct items from a list.
- You can do aggregation operatations.
- And many more.
Selecting Data with LINQ
In today’s episode, let’s look at some selection mechanisms in LINQ. For our explorations, I’ll use this list of ‘Student` objects. Create a new .NET console application and copy the code below into Program.cs
to use as your starting point.
using System; using System.Collections.Generic; public class Student { public int Id { get; set; } public string Name { get; set; } public int Age { get; set; } public string Major { get; set; } public Student(int id, string name, int age, string major) { Id = id; Name = name; Age = age; Major = major; } public override string ToString() { return $"ID: {Id}, Name: {Name}, Age: {Age}, Major: {Major}"; } } public class Program { public static void Main() { List<Student> students = new List<Student> { new Student(1, "Alice", 20, "Computer Science"), new Student(2, "Bob", 22, "Mathematics"), new Student(3, "Charlie", 21, "Physics"), new Student(4, "Diana", 23, "Biology"), new Student(5, "Edward", 20, "Chemistry") }; foreach (var student in students) { Console.WriteLine(student); } } }
Let’s start with something basic — selecting the entire dataset, as is.
// Select ALL items with LINQ // 1. Query sytnax var allStudents = from student in students select student; // 2. Method syntax var students2 = students.Select(student => student);
Above, you can see two different styles of selecting data using LINQ, first using the query syntax and secondly, using the method syntax. Next, instead of selecting the student object as a whole, how can we select just the names of each student?
// Query syntax var names = from student in students select student.Name; foreach (var name in names) { Console.WriteLine(name); } // Method syntax var names2 = students.Select(student => student.Name).ToList(); foreach (var name in names2) { Console.WriteLine(name); }
Above, you can two different ways of getting the student names. Also, did you notice the auto-completion that your editor provided while typing that query out? LINQ is smart enough to provide us with compile-time assistance in writing our queries.
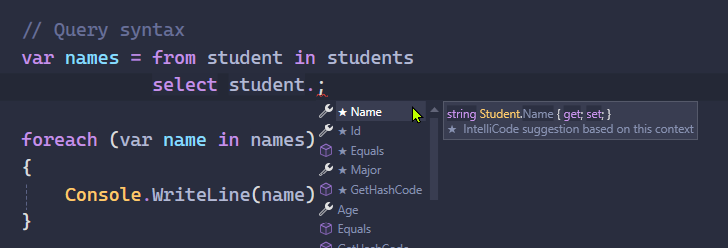
Let’s round off this article in our series by select some of the student data and capture them as new anonymous objects. Please keep in mind that this is merely to illustrate that you can project data into other objects and not a discussion on what anonymous objects are and whether or not you should use them.
var persons = students.Select(student => new { student.Name, student.Age }).ToList(); foreach (var person in persons) { Console.WriteLine(person); }
Here, I was able to pick out just the names and ages of students and store them as new objects.
Conclusion
In this installment, we looked at how we can select data from datasets via LINQ. In the next one, we’ll look at organizing data by sorting them in various ways. Stay tuned.